Online (remote) presentation
The SDK enables presenting a claimed mDoc to a web application for verification via a remote (online) presentation workflow as per ISO/IEC 18013-7:2024 and OID4VP .
To build a step-by-step implementation of this workflow, give the Online presentation tutorial a try.
Sequence diagram
Detailed steps
Ensure your wallet application can be invoked by the verifier application authorization endpoint
During online presentation workflows, verifier web applications invoke matching wallet applications using an Authorization endpoint URI. The wallet application uses the Authorization endpoint URI to retrieve a request object, which details what information is requested for verification.
This URI can be generates by the verifier in one of the following ways:
-
HTTP link (universal link ): This URI would only invoke known and registered applications, as it is verified against the domain owner before launching the application.
If your wallet application is expected to interact with verifier applications that are using HTTP links, you must ensure that the application domain is properly registered with these verifier applications in advance.
-
Custom URI scheme : This URI would invoke any application that is configured to handle this specific custom URI.
If your wallet application is expected to interact with verifier applications that are using custom URI schemes, you must configure your wallet application to handle these specific custom URI schemes. You can see an example for such configuration in our Online presentation tutorial.
Depending on the workflow, the verifier web application will embed the authorization endpoint URI either in a deep-link (for same-device workflows) or a QR code (for cross-device workflows).
Retrieve a request object
Once your wallet application is invoked by the authorization endpoint URI, it must retrieve the request object from the verifier application. This object details:
- What credentials are required.
- What specific claims are required from these credentials.
- What verification server (for example, a MATTR VII verifier tenant) to interact with.
To achieve this, your application must call the SDK’s createOnlinePresentationSession method with the authorization endpoint URI as a parameter, as shown in the following example:
func createOnlinePresentationSession(
authorisationRequestUri: String,
requireTrustedVerifier: Bool = false
) async throws -> OnlinePresentationSession
authorisationRequestURI
: This is the authorization endpoint URI that was retrieved either from the deep-link (same-device workflow) or QR code (cross-device workflow).requireTrustedVerifier
: This determines what verifiers the SDK will accept verification requests from:- When set to
true
the SDK will only accept requests from verifiers that are included in the SDK’s trusted verifiers list. - When set to
false
the SDK will accept requests from any verifier.
- When set to
Adding trusted verifiers
To add a trusted verifier your application should call the SDK’s addTrustedVerifierCertificates method, passing the verifier’s CA certificate as a parameter:
func addCertificate(certificate: String) {
do {
_ = try mobileCredentialHolder.addTrustedVerifierCertificates(certificates: [certificate])
} catch {
print(error.localizedDescription)
}
}
Display request object to user
The createOnlinePresentationSession method uses the authorization endpoint URI to interact with the verifier application and retrieve the request object. This information is returned as an instance of OnlinePresentationSession :
var matchedCredentials: [(request: MobileCredentialRequest, matchedMobileCredentials: [MobileCredentialMetadata])]?
let verifiedBy: OnlinePresentationSession.VerifiedBy
matchedCredentials
: Indicates what information was requested by the verifier for verification and what existing credentials include that information. See below for the detailed structure of this instance property.verifiedBy
: Indicates the verification method used to validate the verifier:certificate
: By its CA certificate.domain
: By its domain.
Your application can now parse this object and display to the user:
- What information is requested for verification.
- What existing credentials match the requested information.
- What claims from these existing credentials will be shared with the verifier.
Then, your wallet application should include a UI element for the user to consent sharing the information with the verifier:
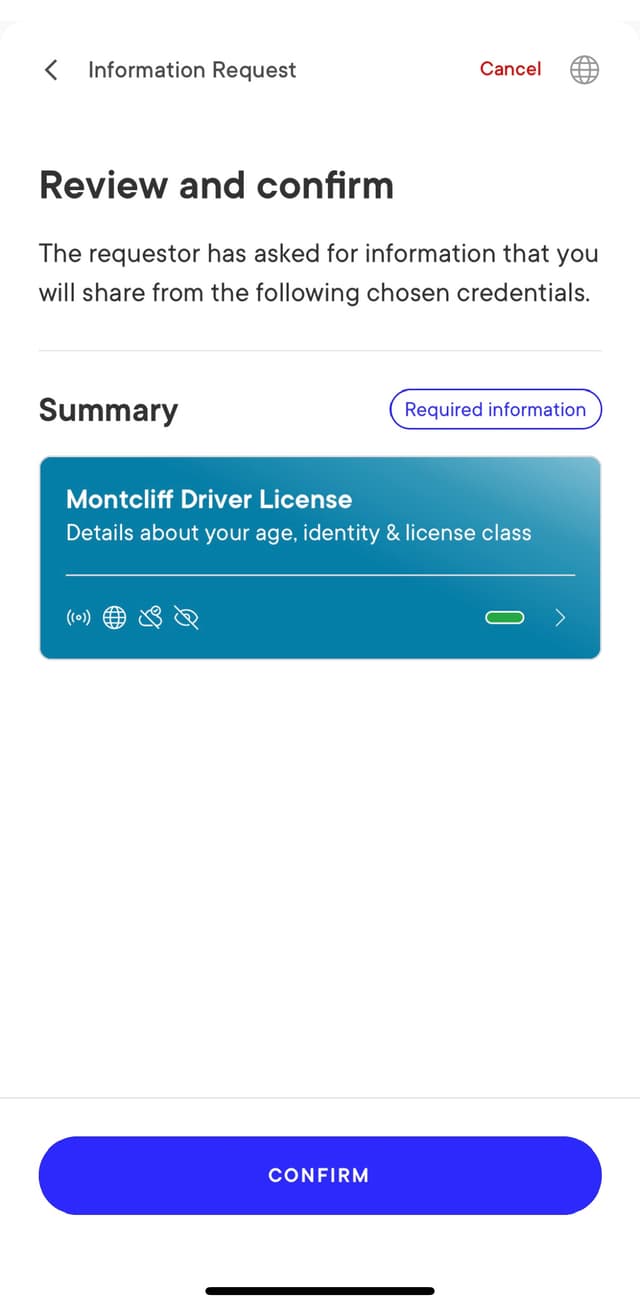
UX Considerations
The MATTR GO example app splits this information into two screens:
- The first screen displays what information is requested for verification.
- Once the users choose to continue, a second screen is displayed with the matching credentials and a consent button.
You can choose to follow a similar approach or combine all information into a single view, depending on your UX/UI preferences.
Whatever approach you choose, it is recommended to create a consistent UX by creating a single view that is used for both the online and proximity presentation flows.
matchedCredentials
structure
The
matchedCredentials
instance property is an array which includes a dictionary of the following structures:
-
request
: This is aMobileCredentialRequest
structure that defines the information requested for verification:MobileCredentialRequest structurevar docType: MobileCredentialDataTypes.DocType let namespaces: [MobileCredentialDataTypes.NameSpace : [MobileCredentialDataTypes.ElementID : MobileCredentialDataTypes.IntentToRetain]]
docType
: Indicates the requested credential type.namespaces
: This is a dictionary of namespaces that are requested for verification. Eachnamespace
is itself a dictionary of:ElementID
: Requested claim identifier.IntentToRetain
: Boolean indicating whether or not the relying party will retain this claim’s value after the verification process is completed.
-
matchedMobileCredentials
: This is aMobileCredentialMetadata
structure that includes the metadata of stored credentials that include the information included in the correspondingMobileCredentialRequest
:matchedMobileCredentials structurelet branding: Branding? let claims: [MobileCredentialDataTypes.NameSpace : [MobileCredentialDataTypes.ElementID]]? let docType: String let id: String let issuerInfo: IssuerInfo let validityInfo: Validity
branding
: Information and links to graphic assets that should be used by your wallet application to render this credential.claims
: This is a dictionary of namespaces that are included in the credential and the identifiers (ElementID
) of claims that are requested for verification from this credential.doctype
: Credential’s type.id
: Unique identifier of this credential.issuerInfo
:commonName
: Issuer’s common name, matching its certificate. This can be used to display the credential issuer’s human readable name to the user.trustedIssuerCertificateId
: Issuer’s IACA certificate.
validityInfo
:validFrom
: Indicates the date when this credential becomes valid.validUntil
: Indicates the date when this credential expires.
The
MobileCredentialMetadata
structure does not include any of the credential claims or verification status. If you want to display this information to the user as part of the verification workflow, use the getCredential method with the credential’sid
to retrieve this information as an instance of MobileCredential . You can then parse this structure and display any required information to the user.
Send response to verifier application
When the user consents to sharing the displayed information with the verifier, your wallet
application must call the SDK’s
sendResponse
method to share the selected credentials with the verifier:
func sendResponse(
credentialIds: [String],
autoRedirect: Bool = true
) async throws -> String?
credentialIds
: Use this array to include the identifiers of all credentials that should be included in the response to the verifier.autoRedirect
: Controls the redirect behavior for same-device workflows:- When set to
true
, the user is automatically redirected to the configured redirect URI (returned asString
by thesendResponse
method). - When set to
false
, your application can manually redirect the user by retrieving the redirect URI from theString
returned bysendResponse
method.
- When set to
UX Considerations
- The SDK’s
sendResponse
method requires user authentication if the user has not authenticated in the last 30 seconds. To enable users to authenticate via Face ID you must add theNSFaceIDUsageDescription
attribute to the application’sinfo.plist
file. - Some verification requests might include more than one credential. Furthermore, the user might have more than one credential that matches the verification request. Your wallet application should therefore offer a UI to enable the user to select what credential(s) to share in the response.
Continue the interaction with the web application
UX Considerations
Once the response is sent, the interaction with the website will continue in accordance with the workflow:
- Same-device workflows: The user is redirected to the web application in their default mobile browser, where the verifier web application should display verification results and allow them to continue the interaction.
- Cross-device workflows: The web application will display the verification results on the desktop browser where the user started the interaction. You might choose to indicate that to the user on their wallet application view, recommending they return to their desktop browser to continue the interaction.